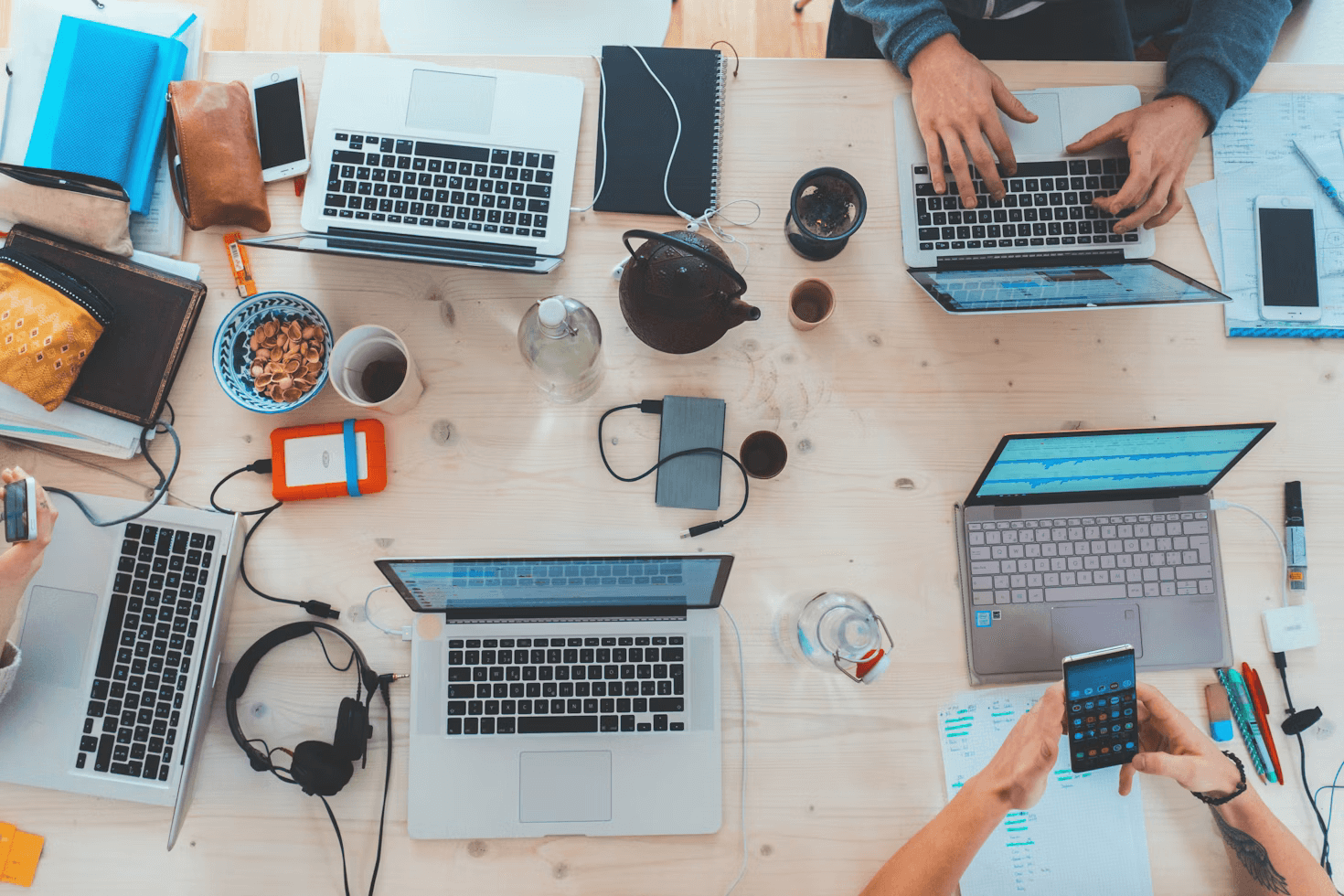
Mastering API Development: Best Practices for Building Robust APIs
APIs (Application Programming Interfaces) are the backbone of modern software development, enabling different applications and services to communicate seamlessly. Building robust and efficient APIs is crucial for ensuring your applications interact smoothly and perform optimally. Here’s a guide to best practices for developing high-quality APIs.
1. Design with the User in Mind
Understand Requirements: Start by understanding the needs of your API users. Gather requirements from stakeholders and consider the different use cases your API will support.
Consistent Design: Use consistent naming conventions, data formats, and structures across your API. This makes it easier for developers to understand and use your API effectively.
2. Use RESTful Principles
Resource-Based: Design your API around resources rather than actions. Use standard HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources.
Stateless Operations: Ensure that each API request contains all the information needed for processing. Avoid relying on server-side sessions, which can reduce scalability.
3. Ensure Security
Authentication: Implement robust authentication mechanisms, such as OAuth 2.0 or API keys, to control access to your API.
Encryption: Use HTTPS to encrypt data transmitted between clients and your API, protecting sensitive information from unauthorized access.
4. Provide Comprehensive Documentation
Detailed API Docs: Create clear, detailed documentation that includes endpoints, request/response formats, authentication methods, and example use cases.
Interactive Documentation: Consider using tools like Swagger or Postman to provide interactive documentation, allowing developers to test API endpoints directly.
5. Implement Error Handling and Versioning
Error Responses: Provide meaningful error messages and status codes to help users diagnose and resolve issues. Use standardized error formats for consistency.
Versioning: Implement versioning in your API (e.g., /v1, /v2) to manage changes and ensure backward compatibility. This allows you to introduce updates without breaking existing clients.
6. Optimize Performance
Caching: Use caching mechanisms to reduce latency and improve response times. Consider implementing server-side caching or using a CDN for static assets.
Rate Limiting: Implement rate limiting to prevent abuse and ensure fair usage of your API resources.